Remember that feeling of accomplishment when you finally mastered the basics of Active Record? You could create, read, update, and delete data with ease. But what about relationships between your models? That’s where the real power of Active Record shines. In this installment of Hero of the Rails, we’ll dive into Active Record associations, unlocking the secrets to building complex applications with seamless data connections.
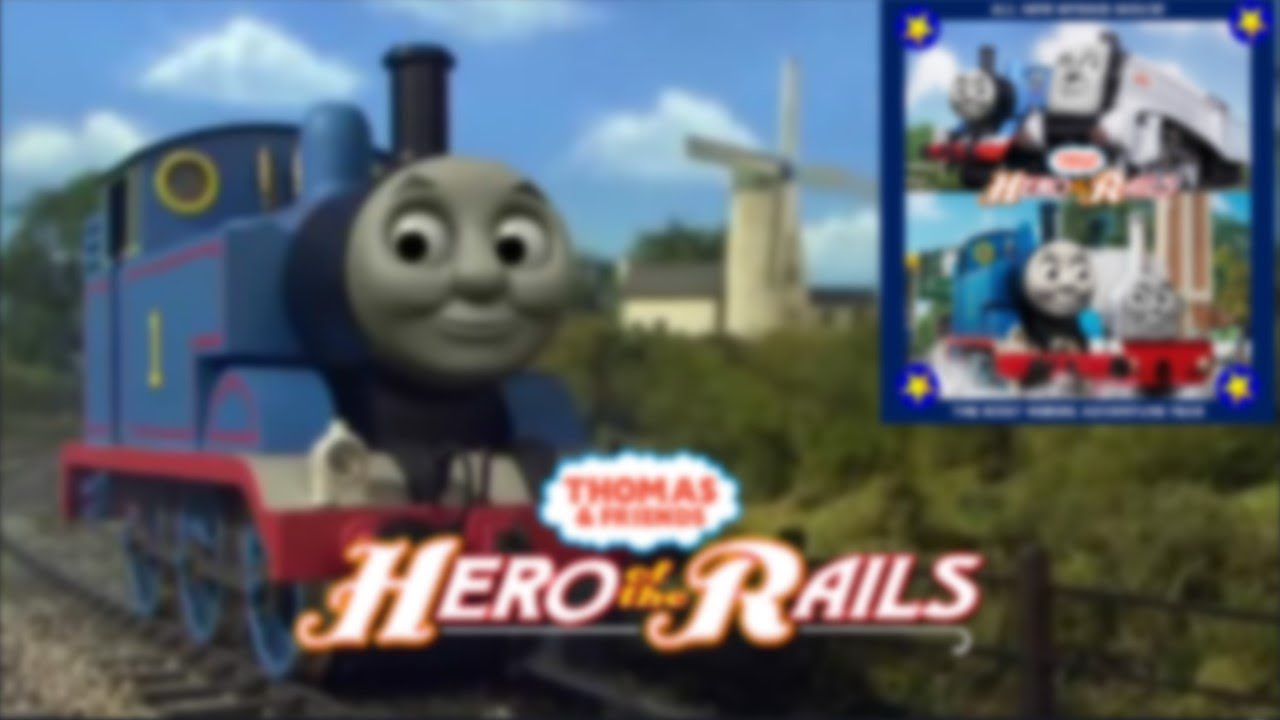
Image: www.youtube.com
Picture this: You’re building an e-commerce site with products and users. Each user can have multiple orders, and each order contains many products. How do you manage this intricate network of data? That’s where Active Record associations come into play, providing a clean and efficient way to define relationships between your models.
Understanding Active Record Associations
Defining Relationships
Active Record associations define how your models relate to each other. You can use them to create various types of connections, such as:
- One-to-one: One record in one model corresponds to exactly one record in another model. Think of a user having a single profile.
- One-to-many: One record in one model can have multiple related records in another model. A user can have multiple orders, for example.
- Many-to-many: Records in both models can have multiple relationships with each other. A product can be in multiple orders, and an order can contain multiple products.
The Power of Convenience
Active Record associations go beyond simply defining relationships. They also provide powerful convenience methods for accessing and manipulating associated data. For instance, you can:
- Fetch associated records: Instead of writing complex queries, you can simply call methods like
user.orders
orproduct.categories
to retrieve related data. - Create and update associated records: Associations simplify creating new records related to existing ones, like adding a new product to an order with
order.products << product
. - Manage dependencies: Active Record associations ensure data integrity by automatically handling cascading deletes, ensuring that related data is appropriately managed.
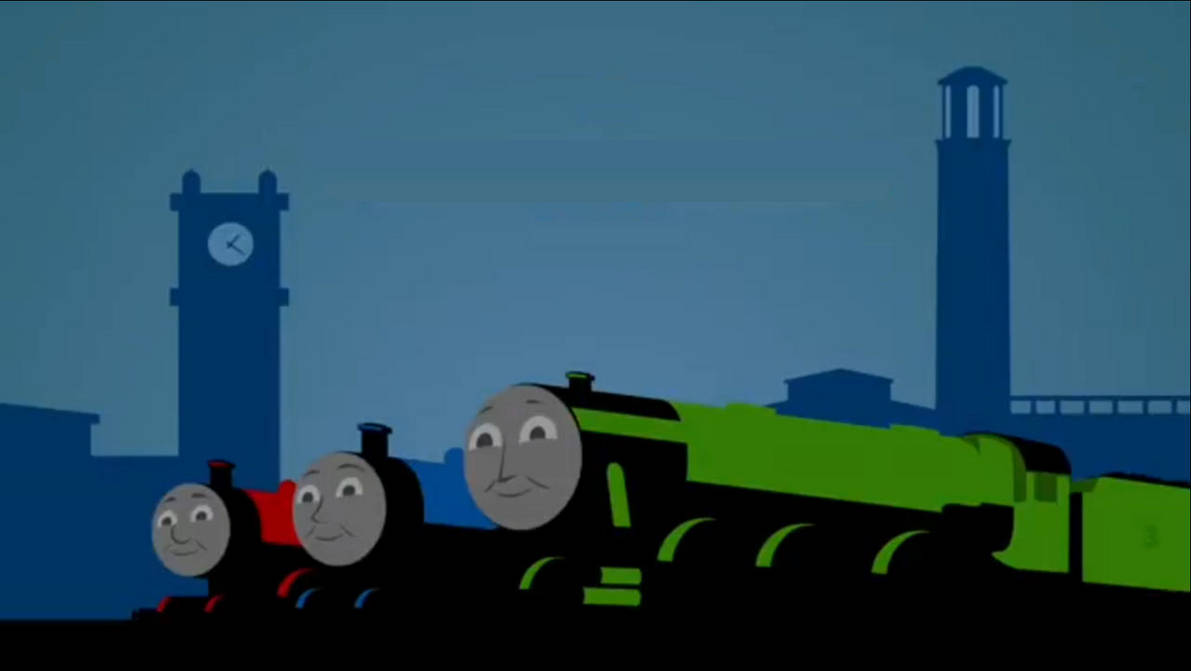
Image: www.deviantart.com
Exploring Association Types
Belongs_to and Has_many
The most common association types are belongs_to
and has_many
. These define one-to-many relationships, where one model "belongs to" another, and the other model "has many" of the first.
Let's consider our e-commerce example. We have a User
model and an Order
model. Users have orders, and orders belong to users. We can establish this relationship with:
class User < ActiveRecord::Base has_many :orders endclass Order < ActiveRecord::Base belongs_to :user end
Now, a User
object can access its orders using user.orders
, and an Order
object can access its user through order.user
.
Has_one
Similar to has_many
, has_one
signifies a one-to-one relationship. Imagine a User
model and a Profile
model, where each user has a single profile.
class User < ActiveRecord::Base has_one :profile endclass Profile < ActiveRecord::Base belongs_to :user end
With this definition, a User
can access its associated Profile
through user.profile
.
Has_and_belongs_to_many
For many-to-many relationships, we use has_and_belongs_to_many
. Let's revisit our e-commerce example with products and categories. A product can belong to multiple categories, and a category can have multiple products.
class Product < ActiveRecord::Base has_and_belongs_to_many :categories endclass Category < ActiveRecord::Base has_and_belongs_to_many :products end
Active Record automatically creates a join table named categories_products
to manage the many-to-many relationship. This table stores a unique entry for each product-category combination, allowing you to access all products belonging to a category using category.products
and all categories related to a product using product.categories
.
Tips and Tricks
Optimize for Performance
When dealing with complex associations, performance can become a concern. Consider using techniques like eager loading:
User.includes(:orders).where(name: "Alice")
By including orders
in the query, all orders for Alice are retrieved in a single database call, avoiding multiple queries and improving performance.
Embrace Nested Attributes
Nested attributes streamline the creation and update of related records. Imagine creating a new order for a user with multiple product items. You can use nested attributes to create all these records in one go:
order = Order.new(user_id: 1, products_attributes: [ name: "Product A", price: 10 , name: "Product B", price: 20 ])
This simplifies the process of managing complex relationships, saving you time and effort.
FAQs
Q: What is the difference between has_many
and has_one
?
A: has_many
defines a one-to-many relationship, where one record can have multiple related records. has_one
signifies a one-to-one relationship, where one record is associated with only one other record.
Q: Where can I find more information about Active Record associations?
A: The official Rails documentation is your go-to resource for detailed explanations, examples, and advanced concepts. You can find it at https://guides.rubyonrails.org/association_basics.html.
Q: How can I troubleshoot issues related to associations?
A: When facing association troubles, utilize your Rails console to inspect objects and relationships. Leverage debugging tools like pry to step through code and examine data flow.
Hero Of The Rails Part 7
Conclusion
Active Record associations are a cornerstone of Rails development, enabling you to create complex applications with relational databases. By mastering these relationships, you'll unlock the power of Active Record, making your code more elegant, concise, and efficient.
Are you ready to explore the world of Active Record associations and elevate your Rails skills? If so, explore the numerous resources available online, experiment with different relationships, and don't hesitate to seek help from the vibrant Rails community!